Field based custom validation is to validate a field with in the same item based on the value of another field. This validation will only kick in after you save the item. For example you want to make couple of fields mandatory based on a check box selection. you can use the below code to achieve it.
Custom Validation code
using Sitecore.Data.Validators;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace Foundation.SitecoreExtensions.Validators
{
public class CustomRequiredFieldsValueBasedValidation : StandardValidator
{
public override string Name => "Required";
protected override ValidatorResult Evaluate()
{
//Get Template Id's for which this validation applies
var templateIds = this.Parameters["TemplateIds"].Split('|');
if (templateIds.Any() && templateIds.Contains(this.GetItem().TemplateID.ToString()))
{
var field = this.Parameters["FieldId"];
//Check if the field parameter exists
if (!string.IsNullOrEmpty(field))
{
if(field.Split('|').Length == 2)
{
var fieldId = field.Split('|')[0];
var fieldExpectedValue = field.Split('|')[1];
//Compare the expected value with Item Expected Value
if(this.GetItem().Fields[fieldId]?.Value != fieldExpectedValue)
{
if (!string.IsNullOrEmpty(this.ControlValidationValue))
{
return ValidatorResult.Valid;
}
}
}
}
this.Text = this.GetText("Field \"{0}\" must contain a value.", this.GetFieldDisplayName());
return this.GetFailedResult(ValidatorResult.CriticalError);
}
return ValidatorResult.Valid;
}
protected override ValidatorResult GetMaxValidatorResult()
{
return this.GetFailedResult(ValidatorResult.Error);
}
}
}
Create a new Field Rules item in Sitecore under Validation Rules as shown below
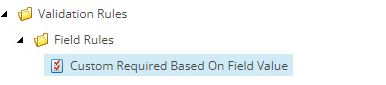
and specify
Type pointsto your custom class and
Parameters
TemplateIds for which this rule applies, multiple templates can be separated by |.
FieldId Source field for validation and the value to validate (value is specified after |)
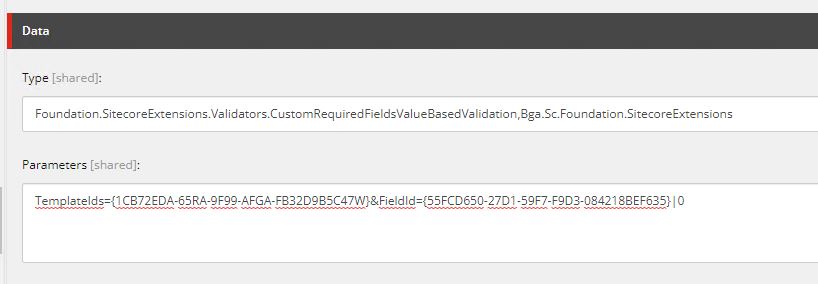